Introducing FastAPI
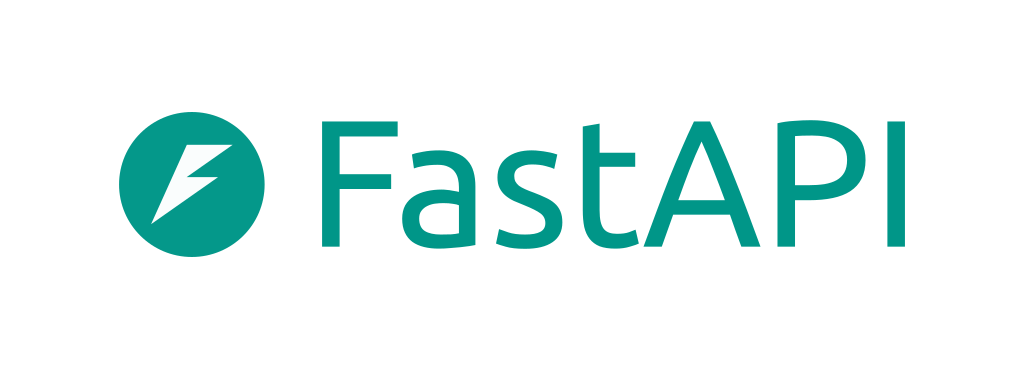
Introduction
Developing APIs can be painful if we don’t know where to start. As I’ve always been a fan of Python, it is my first choice for development. It has a great number of frameworks and libraries supporting web development (e.g., Django, Flask, etc.). This time I would like to introduce a super-fast and easy-to-use framework which I’ve been using for a couple of years now: FastAPI.
FastAPI
FastAPI is a high-performace web framework for creating APIs with Python. It is light and easy to start, we can create a simple API with just a few lines of code
from typing import Optional
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"Hello": "World"}
Start a server by using uvicorn main:app –reload
uvicorn main:app --reload
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
INFO: Started reloader process [28720]
INFO: Started server process [28722]
INFO: Waiting for application startup.
INFO: Application startup complete.
As can be seen above, it is so simple and fast to develop which is suitable for nowadays’ rapid demands for development. Additionally, regardings its performance it is on par with NodeJS and Go, Benchmarks.
Not only it has incredible speed, but also comes with an auto-generated interactive API document (powered by swagger-ui) which users can input parameters and validate outputs with ease.
And my favourite part is that it works perfectly with Pydantic. It just makes data validation and setting management so much fun to handle with proper structures.
from typing import Any, Dict, Optional
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class ResponseModel(BaseModel):
status: int
data: Optional[Dict[str, Any]] = {}
@app.get("/")
def read_root() -> ResponseModel:
res = {
status: 1,
data: {"Hello": "World"}
}
return ResponseModel(**res)
Summary
When it comes to meeting deadline and avoiding working overtime, FastAPI never disappoints me and my teammates. It is great for developing APIs as proof of concept or even for production. I personally recommend this framework for everyone to give it a try. It is awesome.